Thursday, December 28, 2006
How to use Dynamic Object invocation in Rails for Models (String to Model conversion)
-------------
You have String value of the model (model name) and you want to dynamically invoke methods on this model. This comes handy when you want to parse controller/action and do some neat nifty tricks.
Solution
-----------
model_name = "invoice"
model_name_capital = model_name.capitalize # => this creates "Invoice"
@object = Object.const_get(model_name).find(:all) # => This is equivalent to Invoice.find_all
Wednesday, December 27, 2006
Ruby 4 Lines of Web service/SOAP Code Magic
require 'soap/wsdlDriver'
factory = SOAP::WSDLDriverFactory.new("http://api.google.com/GoogleSearch.wsdl")
service = factory.create_rpc_driver
@service_output = service.doGoogleSearch("xxxxxxxxxxxxxx", "Ajuby", 0, 10, "false", "", "false", "", "latin1", "latin1")
That's it.. @service_output returns what I call it as "Ruby's intelligent data structure" which you can use to get respective data output
e.g.
- Results: <%=@service_output.estimatedTotalResultsCount%>
- searchTime: <%=@service_output.searchTime%>
Note: Above "xxxxxxxxxxxxx" is the api key that you need to obtain from Google to use their web services, its less than a minute job to get that from google.
Now there is a better and more modular way of writing above code using ActionWebService
where you can manage API's to access outgoing SOAP calls more effectively.
Monday, November 27, 2006
XSD to WSDL Conversion
# Also Christian Weyer's jWSCF for Eclipse
# ObjectWeb's Celtix ESB's xsd2wsdl tool
Surprisingly first 2 generates different type of WSDL for same XSD.
Tuesday, October 10, 2006
Legends/Great Programmers answer simple but important questions..
I liked the question "If you had three months to learn one relatively new technology, which one would You choose?". Answers to this question can project one vital thing "What is the interesting technology/concept or thing which you would see helping technology future"
Monday, October 09, 2006
Java String replace: java.lang.IndexOutOfBoundsException: No group 2
If you see java.lang.IndexOutOfBoundsException: No group 2 , when you are doing <String>.replaceFirst(<source string>, <target string>) or <String>.replaceAll(<source string>, <target string>).
Reason
You possibly have "$" character in your target string.
Solution
Add java.util.regex.Matcher.quoteReplacement(<target string>), before you apply replace function.
Bug reported on Sun site by someone.
Thursday, October 05, 2006
OpenAppDotOrg Toolkit hits 100 downloads!!.
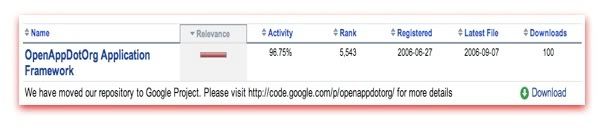
Our Ruby/Rails based open source project OpenAppDotOrg finally hits 100 downloads on sourceforge . Groovy!!... See the captured snapshot of that moment on the left. This is just a beginning, we have a long way to go and I will come back to toast 1,000.....of downloads.
Current release 0.2515 includes, things like
- Common user services (sign up/email support/forgot password etc)
- User access control
- Company/User relationship
- First open source Feed management module
- More about the project can be found on our wiki
- Download the latest version from sourceforge
- Our community on Google groups and openapp forum
Tuesday, October 03, 2006
Rails Association example: has_many relationship
Once you start with this , you can sense the power of this meta modeling techique which turns your business application/model building into a snap.
I will write up more going deeper into complex techniques of Rails association, meanwhile few interesting sites explain this as follows
Friday, August 18, 2006
Ruby/Rails tricks and tips with ActiveRecord
- Write a installer application in rails which will take database username, password and database name
- Creates brand new database
- Populate the database with schema and reference data.
- Updates database.yml file
- Reinitialize the rails activerecord connection context, so that user does not have to restart the application
# Create new database
ActiveRecord::Base.connection.create_database("rex_boy") #rex_boy new database name.
# Load new database with schema and data.
.........This you can do either by using writing Rake migrate tasks or manually write SQL's to load the schema and data, 1000's of ways to do this. I did using creating using migrations and invoking rake command from rails.
# Read and update the database.yml file with newly created database
## Read the Yml and update with new database name
@config = YAML::load(File.open("#{RAILS_ROOT}/config/database.yml")) @config['development']['database']= "rex_boy"
## Now update the yml file back. basically write the database.yml back from @config object
File.open( "#{RAILS_ROOT}/config/database.yml", 'w' ) do |f| f << @config.to_yaml
# Now the interesting part to reintialize ActiveRecord connection context without restarting the server (Apache or WEBrick or Lighttpd..)
ActiveRecord::Base.configurations = @config ActiveRecord::Base.establish_connection ActiveRecord::Base.connection
This should get you very fancy in terms of building rails based installer application.
Thursday, August 10, 2006
Framework comparison
Friday, August 04, 2006
Rails based framework for Java. Co-existence or contender?
# Trails: domain driven development framework in the spirit of Ruby on Rails or Naked Objects. Uses Spring, Tapestry, and Hibernate. Probably this one has a long way to go. Not too much happening in this area.
# Sails: Purely framework designed by keeping Rails in mind. Have a download section.It would be interesting to download and play with it.
# Grails: Sounds the closest one to rails, including controller/model generation, scaffolding etc, its written in Groovy
# JBoss Seam: Probably most active and adopted project as compared to above. Seam uses EJB3 and Hibernate (both, I wonder why?. Probably things around Transaction support and things like heavy weight vs light weight components usage).
I will keep watching this space and add if i run into any other puppy who wants to be or do things like rails in Java.
Friday, June 09, 2006
Quick view on Computer Software/Programming Languages History
Apache would not start if Skype is running
- System Log: "The Apache2 service terminated with service-specific error 1." ; Arghh!! so helpful ;)
- Application Log: "The Apache service named reported the following error:
>>> Unable to open logs" , "The Apache service named reported the following error:
>>> no listening sockets available, shutting down . "
Now the last message is little useful and it says that there is something already listening/running on port 80/443 , But hunting down was tough!. Because I don't have any other web server running. Root cause is "Skype"
Go to Skype->Tools->Options->Connection-> Uncheck "use 80 and 443 for incoming connection"
Who would ever thing my IM will waste my time, where as IM's are supposed to be productivity improvement tools :) , Anyways a good find and I hope it will be useful for others too. BTW No complains for skype, its a great tool.
Tuesday, June 06, 2006
Funky Browser based ruby shell
require 'abracadabra'
=>true
Now I don't think anyone has written gem called abracadabra yet. but this shell is cool, very handy. I tried quick String manipulation test, it works!
Monday, June 05, 2006
Is MySpace becoming IPOD for social community business platform
- SingleStat.us: Its a funny concept, but works for lot of singles or people with old crush. Techcrunch says "Want to know when that cute friend of yours breaks up with her boyfriend....a service that let’s you type in anyone’s Myspace profile and be notified by email if their relationship status changes".
- Nooz: Interactive news service aimed for MySpace users, to start with.
- and lot many...
So the question of the day: Is MySpace becoming IPOD for social community business platform. Like IPOD did, people made lots of dough by spinning their business around IPOD, concepts around IPOD. Now MySapce universe is emerging in a way, it's helping lots of Web2.0 startups to grow.
Monday, May 29, 2006
Buttonator....
Friday, May 19, 2006
Ruby Java Bridge is here
Thursday, May 11, 2006
How Ruby can be next generation business building block
- As Martin fowler said "As a result it certainly seems to me that certain languages are more suited to in-language DSL than others. Seeing lisp and smalltalk I concluded that the more suitable languages were minimalist ones with a single basic idea that's deeper and simpler than traditional languages (function application for lisp, objects and messages for smalltalk). But Ruby is more conventional and a much bigger language than these two, yet is still suitable for in-language DSLs."
- Anothe good read on Metaprogramming idea of Ruby [pdf].
- How to create DSL using Ruby
What does Rails on Apache mean?
Assuming you have a running rails application on WEBrick (which comes with rails), its 5 mins job to have rails up and running on Apache. You will need following things.
Remember You do not need FastCGI at all to run rails on Apache. .
Every rails application created is Apache deployable ready (that sounds so good!!), you don't need anything extra, neither FastCGI or Mongrel. You will need these 2 puppies for some other reasons that I will point out soon.
# Apache HTTP server
# Rails application
2 step process
- Open Apache httpd.conf under
- Add following at the very end and restart Apache
Listen 8027
<VirtualHost 127.0.0.1:8027>
DocumentRoot [railsapppath]/public/
<Directory [railsapppath]/public/>
Options ExecCGI FollowSymLinks
AddHandler cgi-script .cgi
AllowOverride all
Allow from all
Order allow,deny
</Directory>
</VirtualHost>
Or another better way is to (Use Apache Alias technique) add following in your httpd.conf
Alias /railsappname [railsapppath]/public
<Directory [railsapppath]/public>
Options ExecCGI FollowSymLinks
AllowOverride all
Order allow,deny
Allow from all
</Directory>
- Open public/.htaccess file and add "RewriteBase /railsappname "
(With above technique you will not be creating virtual host on different port and this kind of setup makes more sense when you have more than one rails component sitting on Apache)
Here
[railsapppath]/public/: is your rails application public folder. You need to point to this directory, else it won't work.
Port 8027: some random port, on which you are asking Apache to run a virtual host for your rails application.
Now just point your browser to http://127.0.0.1:8007/ and you will see the rails running on Apache. How fast was that?. Oh did I ask How fast was that?. Yes now this takes us to our next topic
# Fast CGI: Fast CGI makes ruby run faster on Apache, otherwise by itself ruby is slow on Apache, because it runs native CGI. I will talk on this in detail, in my next blog topic
Thursday, April 13, 2006
Difference between Web 1.0 and Web 2.0
Thursday, March 30, 2006
Ruby LoadError, Quick fix
I just installed RubyFul_Soup[ Ruby port of the hit Python HTML/XML parser Beautiful Soup.] and wanted get started with RadRails , so when I write this line on top
require 'RubyFulSoup' as per the example given I get following error
LoadError: No such file to load -- rubyfulsoup
The thing is when you install any gem using "gem install" , it installs gem under /usr/lib/ruby/gems/1.8/gems , so somehow ruby does not really get where to pick up gems from , best thing to do a quick sanity check regarding your setup , and you can do that by "irb" (Interactive Ruby Shell), just type
bash> irb
irb(main):002:0> require 'RubyFulSoup'
LoadError: No such file to load -- RubyFulSoup
from (irb):2:in `require'
from (irb):2
basically gem installed is "rubyful_soup' and its under /usr/lib/ruby/gems/1.8/gems
and Ruby looks for files under /usr/lib/ruby/1.8/ , So all I did is copied rubyful_soup.rb file from /usr/lib/ruby/gems/1.8/gems to /usr/lib/ruby/1.8/ and also copy htmltools gem files to /usr/lib/ruby/1.8/, then run
irb(main):001:0> require 'rubyful_soup'
=> true
This is a quick fix till I figure out the real solution for this
Wednesday, March 22, 2006
RadRails puts RoR on Web2.0 development Autobahn
What is RadRails, Its a bomb!, its amazing, seriously I was never this excited, when Eclipse happened to Java/J2EE world. Why because it took me more time get Eclipse up and running with my java project and hook tomcat to it , yadda yadda!. But I could count it on on my fingers how long it took me get one simple rails app up and running with built in WEBrick server. It was just less than 5 mins, I had simple customer application with DB read/write/delete running on my browser.
RadRails is written on Eclipse framework, but extremely sexy, in terms producing components. Like rails/ruby/database/subversion support etc.
Some of the cool features
- mvc key bindings : To jump one context of development to another, moving between view (.rhtml ) to app controller faster.
- Generate tabs: All rails generate command on your fingertips
- Console/Tail : Very helpful in AJAX development where you can see data going back and forth between your client and server.
Oh yeah!, did I say how It was this quick , have a look at the screencast , you got to love this stuff!!..
There is a famous say "You need a reason to convince yourself, why you should do different deed", I guess RadRails is my reason to love Ruby/RoR.
So finally I would say "Got RoR!"
Thursday, February 23, 2006
''Home-Sourcing'' vs. Offshoring - Outsourcing - CFO.com
Monday, January 16, 2006
.htaccess usage on Apache doesn't work?, See what you are missing.
Simplest way to implement directory level authorization on Apache HTTP server is to use .htaccess file with password file combinations, You will find How to Implement Authorization on Apache everywhere. But there are couple of things which you will not find in these sites, because they assume that you would have done this before or you know about this already. So let me run thru basic steps and steps which are generally ignored (but very important get this out there)
Basic Steps
- Create .htaccess file with all the directives like AuthType,AuthName,AuthUserFile,Require attributes
- Create password file (as specified in AuthUserFile) using htpasswd command.
- LoadModule auth_module libexec/httpd/mod_auth.so
- AddModule mod_auth.c
Order allow,deny
Deny from all
Satisfy All
- Most important step which makes or brakes this whole exercise
- "AllowOverride none" should be replaced by "AllowOverride AuthConfig"
With above you should be up and running with Apache Basic Auth.